반응형
1. 페이지 이동
화면을 분할하여 한쪽 Pane에는 페이지 내비게이션 버튼을 만들고, 반대쪽에는 실제 페이지를 불러올 Pane을 구성한다.
<MainPage.xaml>
- 4개의 Page 버튼 각각에 이벤트 함수를 추가하여 정의한다.
<Page
x:Class="PageMovement.MainPage"
xmlns:muxc="using:Microsoft.UI.Xaml.Controls"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:PageMovement"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid>
<muxc:TwoPaneView x:Name="MyTwoPaneView" Pane1Length="0.2*" Grid.Row="1" MinWideModeWidth="959" MinTallModeHeight="1263" ModeChanged="TwoPaneView_ModeChanged">
<muxc:TwoPaneView.Pane1>
<Grid x:Name="Pane1Root" Background="Beige">
<ScrollViewer>
<StackPanel x:Name="Pane1StackPanel" Orientation="Vertical" Margin="10,10,10,10" HorizontalAlignment="Center" VerticalAlignment="Center">
<Button x:Name="Mode1" Content="Page1" Width="Auto" Margin="10,10,10,10" Background="White" FontSize="45" Click="Mode1_Click" IsEnabled="True"/>
<Button x:Name="Mode2" Content="Page2" Width="Auto" Margin="10,10,10,10" Background="White" FontSize="45" Click="Mode2_Click" IsEnabled="True"/>
<Button x:Name="Mode3" Content="Page3" Width="Auto" Margin="10,10,10,10" Background="White" FontSize="45" Click="Mode3_Click" IsEnabled="True"/>
<Button x:Name="Mode4" Content="Page4" Width="Auto" Margin="10,10,10,10" Background="White" FontSize="45" Click="Mode4_Click" IsEnabled="True"/>
</StackPanel>
</ScrollViewer>
</Grid>
</muxc:TwoPaneView.Pane1>
<muxc:TwoPaneView.Pane2>
<Grid x:Name="Pane2Root">
<ScrollViewer x:Name="DetailsContent">
<Frame Background="Azure" Grid.Row="1" x:Name="frame" Margin="10,10,10,10" HorizontalAlignment="Center"
VerticalAlignment="Center" Width="1550" Height="940">
</Frame>
</ScrollViewer>
</Grid>
</muxc:TwoPaneView.Pane2>
</muxc:TwoPaneView>
</Grid>
</Page>
<MainPage.xaml.cs>
- 각 버튼을 클릭할 시에 해당 페이지를 Frame으로 불러온다.
using muxc = Microsoft.UI.Xaml.Controls;
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using Windows.UI.Notifications;
using Windows.Data.Xml.Dom;
using Windows.UI.Popups;
using Windows.UI;
using Windows.UI.Core;
using System.Threading.Tasks;
using Windows.Security.Cryptography;
// 빈 페이지 항목 템플릿에 대한 설명은 https://go.microsoft.com/fwlink/?LinkId=402352&clcid=0x412에 나와 있습니다.
namespace PageMovement
{
/// <summary>
/// 자체적으로 사용하거나 프레임 내에서 탐색할 수 있는 빈 페이지입니다.
/// </summary>
public sealed partial class MainPage : Page
{
IStateVariables variables = new StateVariables();
public MainPage()
{
try
{
this.InitializeComponent();
}
catch(Exception e)
{
Console.WriteLine(e.ToString());
}
}
private void TwoPaneView_ModeChanged(Microsoft.UI.Xaml.Controls.TwoPaneView sender, object args)
{
// Remove details content from it's parent panel.
((Panel)DetailsContent.Parent).Children.Remove(DetailsContent);
// Set Normal visual state.
Windows.UI.Xaml.VisualStateManager.GoToState(this, "Normal", true);
// Single pane
if (sender.Mode == Microsoft.UI.Xaml.Controls.TwoPaneViewMode.SinglePane)
{
// Add the details content to Pane1.
Pane1StackPanel.Children.Add(DetailsContent);
}
// Dual pane.
else
{
// Put details content in Pane2.
Pane2Root.Children.Add(DetailsContent);
// If also in Wide mode, set Wide visual state
// to constrain the width of the image to 2*.
if (sender.Mode == Microsoft.UI.Xaml.Controls.TwoPaneViewMode.Wide)
{
Windows.UI.Xaml.VisualStateManager.GoToState(this, "Wide", true);
}
}
}
private void Mode1_Click(object sender, RoutedEventArgs e)
{
frame.Navigate(typeof(BlankPage1));
}
private void Mode2_Click(object sender, RoutedEventArgs e)
{
frame.Navigate(typeof(BlankPage2));
}
private void Mode3_Click(object sender, RoutedEventArgs e)
{
frame.Navigate(typeof(BlankPage3));
}
private void Mode4_Click(object sender, RoutedEventArgs e)
{
frame.Navigate(typeof(BlankPage4));
}
}
}
2. Grid 화면 분할
<BlankPage1.xaml>
- Grid.RowDefinitions 및 Grid.ColumnDefinitions를 이용하여 화면을 분할한다.
- BlankPage2, BlankPage3, BlankPage4도 원하는 화면으로 구성한다.
<Page
x:Class="PageMovement.BlankPage1"
xmlns:muxc="using:Microsoft.UI.Xaml.Controls"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:PageMovement"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="*"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*"/>
<ColumnDefinition Width="*"/>
<ColumnDefinition Width="*"/>
</Grid.ColumnDefinitions>
<Border Grid.Row="0" Grid.Column="0" Background="#CCFFFF" Margin="10,10,10,10">
<StackPanel>
<TextBlock Text="Section1" FontSize="35" HorizontalAlignment="Center" Margin="10"/>
<StackPanel Orientation="Horizontal" Margin="0,150,0,0" HorizontalAlignment="Center">
<TextBlock Text="TextBlock and Rectangle" FontSize="20" HorizontalAlignment="Center" Margin="0,0,20,0"/>
<Rectangle x:Name="Earth_bit" Width="30" Height="30" Stroke="Black" Fill="DarkRed" StrokeThickness="5"></Rectangle>
</StackPanel>
</StackPanel>
</Border>
<Border Grid.Row="1" Grid.Column="0" Background="#CCFFFF" Margin="10,10,10,10">
<StackPanel>
<TextBlock Text="Section4" FontSize="35" HorizontalAlignment="Center" Margin="10"/>
<StackPanel Orientation="Horizontal" Margin="0,150,0,0" HorizontalAlignment="Center">
<TextBlock Text="TextBlock and Rectangle" FontSize="20" HorizontalAlignment="Center" Margin="0,0,20,0"/>
<Rectangle x:Name="Oper_bit" Width="30" Height="30" Stroke="Black" Fill="DarkRed" StrokeThickness="5"></Rectangle>
</StackPanel>
</StackPanel>
</Border>
<Border Grid.Row="0" Grid.Column="1" Background="#CCFFFF" Margin="10,10,10,10">
<StackPanel>
<TextBlock Text="Section2" FontSize="35" HorizontalAlignment="Center" Margin="10"/>
<StackPanel Orientation="Horizontal" Margin="0,150,0,0" HorizontalAlignment="Center">
<TextBlock Text="TextBlock and Rectangle" FontSize="20" HorizontalAlignment="Center" Margin="0,0,20,0"/>
<Rectangle x:Name="Sonar_bit" Width="30" Height="30" Stroke="Black" Fill="DarkRed" StrokeThickness="5"></Rectangle>
</StackPanel>
</StackPanel>
</Border>
<Border Grid.Row="1" Grid.Column="1" Background="#CCFFFF" Margin="10,10,10,10">
<StackPanel>
<TextBlock Text="Section5" FontSize="35" HorizontalAlignment="Center" Margin="10"/>
<StackPanel Orientation="Horizontal" Margin="0,150,0,0" HorizontalAlignment="Center">
<TextBlock Text="TextBlock and Rectangle" FontSize="20" HorizontalAlignment="Center" Margin="0,0,20,0"/>
<Rectangle x:Name="Boom_bit" Width="30" Height="30" Stroke="Black" Fill="DarkRed" StrokeThickness="5"></Rectangle>
</StackPanel>
</StackPanel>
</Border>
<Border Grid.Row="0" Grid.Column="2" Background="#CCFFFF" Margin="10,10,10,10">
<StackPanel>
<TextBlock Text="Section3" FontSize="35" HorizontalAlignment="Center" Margin="10"/>
<StackPanel Orientation="Horizontal" Margin="0,10,0,0" HorizontalAlignment="Center">
<TextBlock Text="TextBlock and Rectangle" FontSize="20" HorizontalAlignment="Center" Margin="0,0,45,0"/>
<Rectangle x:Name="Wireless_bit" Width="30" Height="30" Stroke="Black" Fill="DarkRed" StrokeThickness="5"></Rectangle>
</StackPanel>
<Border Margin="0,50,0,0" Background="#99FFFF">
<StackPanel Orientation="Vertical" HorizontalAlignment="Center">
<CheckBox FontSize="20" Margin="5">check box</CheckBox>
<TextBox FontSize="20" Width="350" Header="ID" PlaceholderText="Input ID" Margin="5"></TextBox>
<TextBox FontSize="20" Width="350" Header="Password" PlaceholderText="Input Password" Margin="5"></TextBox>
<StackPanel Orientation="Horizontal" HorizontalAlignment="Center">
<Button FontSize="20" Margin="15">Login</Button>
<Rectangle x:Name="comm_result" Width="30" Height="30" Stroke="Black" Fill="DarkRed" StrokeThickness="5"></Rectangle>
</StackPanel>
</StackPanel>
</Border>
</StackPanel>
</Border>
<Border Grid.Row="1" Grid.Column="2" Background="#FFCCCC" Margin="10,10,10,10">
<StackPanel>
<TextBlock Text="Section6" FontSize="35" HorizontalAlignment="Center" Margin="10" FontWeight="SemiBold"/>
<Border Background="#FFCCFF">
<TextBlock Name="txtbox" Width="600" Height="430" FontSize="20" LineStackingStrategy="BlockLineHeight" Foreground="#330033" Margin="10,10,10,10">
<LineBreak/>
2022-05-16 17:40:38 Hello World!
<LineBreak/>
2022-05-16 17:41:17 Bye World!
</TextBlock>
</Border>
</StackPanel>
</Border>
</Grid>
</Page>
3. 결과화면
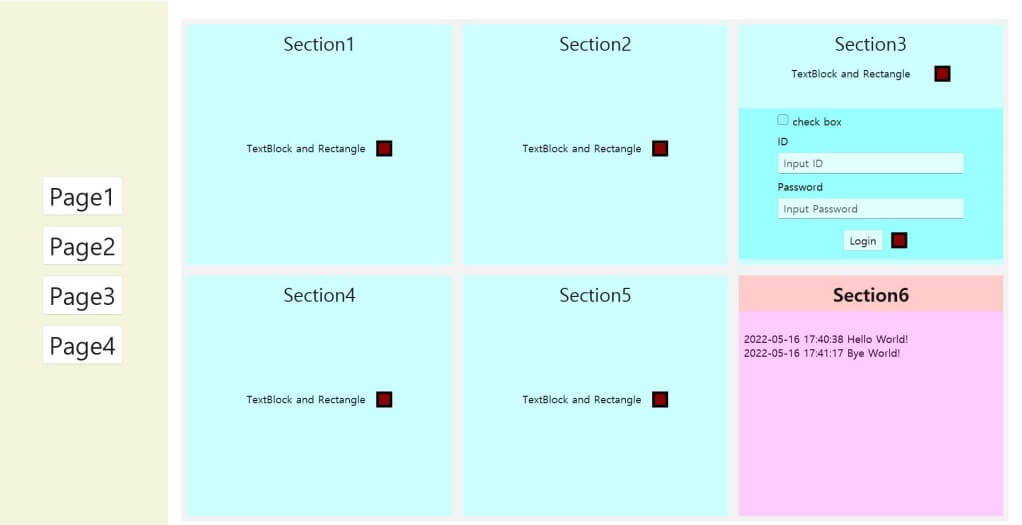
반응형
'프로그래밍 > UWP | WPF' 카테고리의 다른 글
[UWP] 프로그램 실행 시 화면의 크기를 최대화하는 방법 (0) | 2022.08.11 |
---|---|
UWP Grid를 이용한 화면 분할 및 Border를 이용한 Grid 연결 (0) | 2022.05.25 |
UWP 프로그램 실행 시에 랜덤 이미지 보여주기 (0) | 2022.05.25 |
UWP 버튼에 마우스 커서 올리면(mouse hover) 안보임 해결방법 (0) | 2022.05.24 |
UWP(Universal Windows Platform) 예제 프로그래밍 튜토리얼 (0) | 2022.05.09 |
댓글